API - semilattices¶
Classes
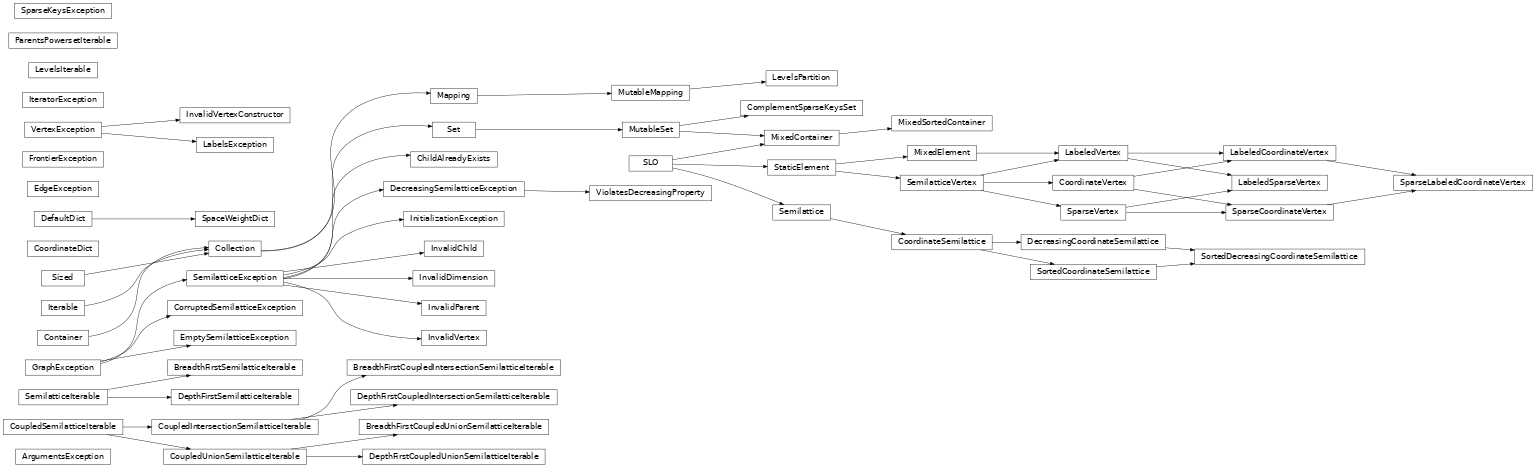
Class | Description |
---|---|
ComplementSparseKeysSet | This is used to handle the sparse_keys (admissible children) of the root. |
CoordinateDict | Sorted dictionary of coordinates. It defaults to zero for missing elements. |
DefaultDict | This is a dictionary which defaults to a prescribed value if a key is missing. |
LevelsPartition | Dictionary for keeping track of subsets of a coordinate semilattice at each level. |
MixedElement | An element whose comparability can change. |
MixedSortedContainer | This is a container where some elements are sorted if assigned a label(s), otherwise are just stored. |
SpaceWeightDict | This is a dictionary that default to 1 for missing elements. |
StaticElement | An element whose comparability cannot change. |
ArgumentsException | |
ChildAlreadyExists | |
CorruptedSemilatticeException | |
DecreasingSemilatticeException | |
EdgeException | |
EmptySemilatticeException | |
FrontierException | |
GraphException | |
InitializationException | |
InvalidChild | |
InvalidDimension | |
InvalidParent | |
InvalidVertex | |
InvalidVertexConstructor | |
IteratorException | |
LabelsException | |
SemilatticeException | |
SparseKeysException | |
ViolatesDecreasingProperty | |
VertexException | |
SemilatticeIterable | Base class defining an iterable for the semilattice. |
BreadthFirstSemilatticeIterable | Breadth first iterable for the semilattice. |
DepthFirstSemilatticeIterable | Depth first iterable for the semilattice. |
CoupledSemilatticeIterable | Base class defining an iterable for two semilattices at the same time. |
CoupledIntersectionSemilatticeIterable | Iterable over the intersection of two semilattices. |
BreadthFirstCoupledIntersectionSemilatticeIterable | Breadth first iterable over the intersection of two semilattices. |
DepthFirstCoupledIntersectionSemilatticeIterable | Depth first iterable over the intersection of two semilattices. |
CoupledUnionSemilatticeIterable | Iterable over the union of two semilattices. |
BreadthFirstCoupledUnionSemilatticeIterable | Iterable over the union of two semilattices. |
DepthFirstCoupledUnionSemilatticeIterable | Iterable over the union of two semilattices. |
LevelsIterable | Iterable over the semilattice vertices by level. |
ParentsPowersetIterable | Iterable over the vertices that are the ancestors defined by the powerset of the parent directions of v. |
SLO | Base object for every object in the module. |
SemilatticeVertex | A vertex that can have multiple parents. |
SparseVertex | A vertex that admit children only in a prescribed set of directions. |
CoordinateVertex | A semilattice vertex that have the concept of directional distance from the root. |
LabeledVertex | Labeled Vertices are provided an optional label(s). |
LabeledSparseVertex | A vertex that is LabeledVertex and SparseVertex . |
SparseCoordinateVertex | A vertex that is CoordinateVertex and SparseVertex . |
LabeledCoordinateVertex | A vertex that is LabeledVertex and CoordinateVertex . |
SparseLabeledCoordinateVertex | A vertex that is LabeledVertex , CoordinateVertex and SparseVertex . |
Semilattice | An (order-) semilattice is a rooted connected directed acyclic graph resembling the structure of a tree, i.e. it has a depth/levels. However, unlike a tree, each vertex can have multiple parents. |
CoordinateSemilattice | A semilattice with pre-defined dimension. |
DecreasingCoordinateSemilattice | A DecreasingCoordinateSemilattice is a semilattice \((\mathcal{S}\,\le)\) that is closed under meets, |
SortedCoordinateSemilattice | A SortedCoordinateSemilattice is a semilattice with Labeled vertices. |
SortedDecreasingCoordinateSemilattice |
Functions
Function | Description |
---|---|
adjacency_mat_eq | Checks equality between two adjacency matrices |
adjacency_mat | Creates an adjacency matrix defining the directed edges (in or out) between vertices and other_vertices |
setLogLevel | Set the log level for all existing and new objects related to the semilattices module |
deprecate | |
cprofile | |
any_kwarg_required | |
default_kwargs | |
exactly_one_kwarg_optional | |
exactly_one_kwarg_required | |
invalid_type | |
optional_kwargs_types | |
require_kwargs | |
required_kwargs | |
valid_type | |
argsort | |
create_lp_semilattice | Create the semilattice of all vertices in the \(\ell^p\) spherical sector of a given norm |
permute | Creates a new semilattice with permuted dimensions |
Documentation
-
semilattices.
adjacency_mat_eq
(mat, other_mat)[source]¶ Checks equality between two adjacency matrices
-
semilattices.
adjacency_mat
(vertices, other_vertices, mat_type=<class 'scipy.sparse.coo.coo_matrix'>)[source]¶ Creates an adjacency matrix defining the directed edges (in or out) between vertices and other_vertices
-
class
semilattices.
ComplementSparseKeysSet
(*args, **kwargs)[source]¶ This is used to handle the
sparse_keys
(admissible children) of the root.Instead of storing the sparse keys of the root, one stores the children of the root which happens implicitely when one tries to remove the child from this data structure. All set operations should be operations that are complements with respect to the set of integers [0, self.max_dim - 1].
-
__init__
(*args, **kwargs)[source]¶ Keyword Arguments: max_dim (int) – maximum dimension of the associated semilattice.
-
add
(key)[source]¶ Adding to the ComplementSparseKeysSet is simply removing from the set, if the key existed in the first place.
-
clear
()[source]¶ Clearing the ComplementSparseKeysSet is is equivalent to removing all elements from
_keys
-
max_dim
¶ Maximum dimension for the complement sparse key set data structure.
-
-
class
semilattices.
CoordinateDict
(obj=None, *args, **kwargs)[source]¶ Sorted dictionary of coordinates. It defaults to zero for missing elements.
We interpret a
key
as a dimension and avalues
as the coordinate in thekey
dimension. A coordinate can be used to identify the position of a vertex in aCoordinateSemilattice
. In particular in this case, each elementkey, value
indicates that a vertex isvalue
steps away in thekey
direction from the root vertex.-
copy
()[source]¶ Creates a copy of the object.
By default, creates a copy that is an instance of
ImmutableCoordinateDict
. Ifimmutable == False
, then it creates an instance ofCoordinateDict
. This is used when creating a neighboring vertex.
-
dims_sorted
¶ Sorted list of dimensions #should be a tuple, but not worth the comptational cost of creating the tuple?
Type: list
-
lp
(p, w=None)[source]¶ Returns the \(l_p\) norm (\(p\geq 1\)) or quasi-norm (\(0\leq p < 1\)) of the coordinates.
For unweighted spaces this is
\[\Vert {\bf c} \Vert_p = \left( \sum_{i=1}^d {\bf c}_i^p \right)^{1/p} \;.\]For weighted spaces this is
\[\Vert {\bf c} \Vert_p = \left( \sum_{i=1}^d {\bf w}_i {\bf c}_i^p \right)^{1/p} \;.\]Parameters:
-
permute
(p)[source]¶ Permutes the keys of the coordinates
Given the naturally ordered coordinates
{0:c[0], 1:c[1], ... )
and the permutationp
, the resulting coordinates will be(0:c[p[0]], 1:c[p[1]], ...)
.Parameters: p (iterable) – permutation of the coordinates
-
stamp
¶ Returns tuple (hashable) of sorted
-
-
class
semilattices.
DefaultDict
(*args, **kwargs)[source]¶ This is a dictionary which defaults to a prescribed value if a key is missing.
-
class
semilattices.
LevelsPartition
(iterable=(), level_attr='l1', level_container_constructor=<class 'set'>, level_container_constructor_kwargs={})[source]¶ Dictionary for keeping track of subsets of a coordinate semilattice at each level.
The
i
’th key corresponds to a SortedSet of all of the vertices of the semilattice in leveli
. It has the interface of adict
as well as of aset
.-
__init__
(iterable=(), level_attr='l1', level_container_constructor=<class 'set'>, level_container_constructor_kwargs={})[source]¶ Parameters: - optional_iterator (iterator) – iterator with initializiation elements
- level_attr (str) – attribute of vertices to use to define the level.
Default is the \(l_1\) norm
l1
. - level_container_constructor (class) – the constructor for the
levels containers. Shold have the
add
,remove
andclear
functions. - level_container_constructor_kwargs (dict) – arguments to be passed to the level container constructor.
-
add
(vertex)[source]¶ Add a vertex to the partition.
It takes the
level_attr
of the vertex and places the vertex in the correct element of the partition
-
discard
(vertex)[source]¶ Remove a vertex from the partition if present
It takes the
level_attr
of the vertex and remove the vertex from the partition
-
get_level
(vertex)[source]¶ Get the container holding elements at the same level of
vertex
Note: Vertex does not have to belong to such level. The properties of vertex are used to get the container that would contain it.
-
remove
(vertex)[source]¶ Remove a vertex from the partition.
It takes the
level_attr
of the vertex and remove the vertex from the partitionRaises: KeyError
– if vertex is missing
-
-
class
semilattices.
MixedElement
(comparable_flag=False)[source]¶ An element whose comparability can change.
Objects of the same class can be comparable or not at different times. Unlike for
StaticElement
, here the comparability of the object can be changed.
-
class
semilattices.
MixedSortedContainer
(*iterator, **kwargs)[source]¶ This is a container where some elements are sorted if assigned a label(s), otherwise are just stored.
All elements are stored in a set (access is O(1)). The elements that are assigned a value to the key
key
are added also to a sorted list(s).-
__init__
(*iterator, **kwargs)¶ Parameters: iterator (iterator) – an iterator with elements initializing the data structure
Keyword Arguments: - label_keys (iterable) – an iterable of label (string) attributes for sorting
- default_label_key (string) – default label
-
discard
(vertex)[source]¶ Remove a vertex from the data structure. Does not Raise exception if missing.
-
get_sorted
(label_key=None)[source]¶ Returns the iterator over the sorted (by default label) labeled vertices.
Warning
It will iterate ONLY over the vertices that have been assigned a label. A warning will be raised if some of the vertices have not been labeled.
-
remove
(vertex)[source]¶ Remove a vertex from the data structure. Raises exception if missing.
Raises: KeyError – if the vertex does not belong to the data structure.
-
sorted
¶ Returns the iterator over the sorted (by default label) labeled vertices.
Warning
It will iterate ONLY over the vertices that have been assigned a label. A warning will be raised if some of the vertices have not been labeled.
-
update_labels
(vertex, **kwargs)[source]¶ Updates the
label(s)
of the vertex.Internally the
MixedElement
function_update_labels
is called in order to make the vertex comparable.
-
-
class
semilattices.
SpaceWeightDict
(*args, **kwargs)[source]¶ This is a dictionary that default to 1 for missing elements.
-
class
semilattices.
StaticElement
(comparable_flag=False)[source]¶ An element whose comparability cannot change.
Within semilattice we use this for objects of the same class that either are comparable between each other or are not. Unlike for
MixedElement
, here comparability is a fixed property.-
__init__
(comparable_flag=False)[source]¶ Parameters: comparable_flag (bool) – whether the object is comparable or not.
-
is_comparable
¶ Whether the object can be compared.
-
-
class
semilattices.
SemilatticeIterable
(graph, QueueConstructor, start_vertex=None, iter_attribute='children')[source]¶ Base class defining an iterable for the semilattice.
Parameters: - graph (
Semilattice
) – semilattice to iterate through - QueueConstructor – queue constructor to be used within the iterator.
This will define whether iteration is breadth or depth first.
See
BreadthFirstSemilatticeIterable
andDepthFirstSemilatticeIterable
for more details. - start_vertex (
SemilatticeVertex
) – vertex from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
-
start_vertex
¶ Starting vertex
Type: SemilatticeVertex
- graph (
-
class
semilattices.
BreadthFirstSemilatticeIterable
(graph, start_vertex=None, iter_attribute='children')[source]¶ Breadth first iterable for the semilattice.
Parameters: - graph (
Semilattice
) – semilattice to iterate through - start_vertex (
SemilatticeVertex
) – vertex from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph (
-
class
semilattices.
DepthFirstSemilatticeIterable
(graph, start_vertex=None, iter_attribute='children')[source]¶ Depth first iterable for the semilattice.
Parameters: - graph (
Semilattice
) – semilattice to iterate through - start_vertex (
SemilatticeVertex
) – vertex from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph (
-
class
semilattices.
CoupledSemilatticeIterable
(graph1, graph2, QueueConstructor, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Base class defining an iterable for two semilattices at the same time.
The intended behavior is similar to the Python function
zip()
, but defining breadth/depth first iterators over the union/intersection of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - QueueConstructor – queue constructor to be used within the iterator.
This will define whether iteration is breadth or depth first.
See
BreadthFirstSemilatticeIterable
andDepthFirstSemilatticeIterable
for more details. - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
CoupledIntersectionSemilatticeIterable
(graph1, graph2, QueueConstructor, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Iterable over the intersection of two semilattices.
The intended behavior is similar to the Python function
zip()
, but defining breadth/depth first iterators over the intersection of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - QueueConstructor – queue constructor to be used within the iterator.
This will define whether iteration is breadth or depth first.
See
BreadthFirstSemilatticeIterable
andDepthFirstSemilatticeIterable
for more details. - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
BreadthFirstCoupledIntersectionSemilatticeIterable
(graph1, graph2, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Breadth first iterable over the intersection of two semilattices.
The intended behavior is similar to the Python function
zip()
, but defining breadth first iterators over the intersection of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
DepthFirstCoupledIntersectionSemilatticeIterable
(graph1, graph2, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Depth first iterable over the intersection of two semilattices.
The intended behavior is similar to the Python function
zip()
, but defining depth first iterators over the intersection of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
CoupledUnionSemilatticeIterable
(graph1, graph2, QueueConstructor, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Iterable over the union of two semilattices.
The intended behavior is similar to the Python function
zip()
, but defining breadth/depth first iterators over the union of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - QueueConstructor – queue constructor to be used within the iterator.
This will define whether iteration is breadth or depth first.
See
BreadthFirstSemilatticeIterable
andDepthFirstSemilatticeIterable
for more details. - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
BreadthFirstCoupledUnionSemilatticeIterable
(graph1, graph2, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Iterable over the union of two semilattices.
The intended behavior is similar to the Python function
zip()
, but defining breadth first iterators over the union of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
DepthFirstCoupledUnionSemilatticeIterable
(graph1, graph2, start_vertex1=None, start_vertex2=None, iter_attribute='children')[source]¶ Iterable over the union of two semilattices.
The intended behavior is similar to the Python function
zip()
, but defining depth first iterators over the union of two semilattices.Parameters: - graph1 (
Semilattice
) – first semilattice to iterate through - graph2 (
Semilattice
) – second semilattice to iterate through - start_vertex1 (
SemilatticeVertex
) – vertex in the first semilattice from which to start the iteration. - start_vertex2 (
SemilatticeVertex
) – vertex in the second semilattice from which to start the iteration. - iter_attribute (str) – attribute of
SemilatticeVertex
to iterate through. Default ischildren
. Alternative can beparents
, to iterate upward towards the root.
- graph1 (
-
class
semilattices.
LevelsIterable
(sl, start_level=0, iter_attribute='_l1_vertices_partition')[source]¶ Iterable over the semilattice vertices by level.
Parameters: - sl (
Semilattice
) – semilattice to iterate through. - start_level (int) – level from which to start.
- sl (
-
class
semilattices.
ParentsPowersetIterable
(v)[source]¶ Iterable over the vertices that are the ancestors defined by the powerset of the parent directions of v. This set of vertices forms a lattice, or ‘hypercube’ of vertices. A sign, indicating that a vertex visited is either an even or odd number of levels away from the original vertex v is also returned. :param v: vertex for which to iterate through parents powerset :type v:
CoordinateVertex
-
semilattices.
setLogLevel
(level)[source]¶ Set the log level for all existing and new objects related to the semilattices module
Parameters: level (int) – logging level
-
class
semilattices.
SLO
[source]¶ Base object for every object in the module.
This object provides functions for storage and logging.
-
class
semilattices.
SemilatticeVertex
(obj=None, *args, **kwargs)[source]¶ A vertex that can have multiple parents.
A
SemilatticeVertex
is an element of a semilattice. There is no limit to the number of vertices that it can contain. Children and parents are stored inSortedDict
.-
children
¶ The children vertices in the semilattice
Type: SortedDict
-
parents
¶ The parent vertices in the semilattice
Type: SortedDict
-
-
class
semilattices.
SparseVertex
(obj=None, *args, **kwargs)[source]¶ A vertex that admit children only in a prescribed set of directions.
-
partial_siblings_count
¶ A counter recording the number of present siblings in each direction.
For direction
d
,partial_siblings_count[d]
is the number of siblings already available, which would be the parents of a child ofself
in thed
direction.Type: Counter
-
-
class
semilattices.
CoordinateVertex
(obj=None, *args, **kwargs)[source]¶ A semilattice vertex that have the concept of directional distance from the root.
CoordinateVertex
can be canonically ordered, because the coordinates are natural number valued and the keys for parents/children are also natural numbers.Keyword Arguments: coordinates (CoordinateDict) – semilattice coordinate where each (key,value)
element indicates that the vertex isvalue
distant from the root in the directionkey
.-
coordinates
¶ immutable dictionary of coordinates
Type: CoordinateDict
-
-
class
semilattices.
LabeledVertex
(obj=None, *args, **kwargs)[source]¶ Labeled Vertices are provided an optional label(s).
Two vertices are comparable if both of them are provided a label (i.e. scalar or anything ‘<’ comparable)
To make sorted data structures robust to the user changing the label(s), the data structure containing the element should be used to update the label of the vertices.
Keyword Arguments: -
data
¶ Dictionary of metadata attached to the vertex. Data metadata are not used as ‘labels’, which define orderings for vertices.
-
default_label
¶ Default Label dictionary for the vertex, used for < = comparison
-
labels
¶ Dictionary of labels for the vertex
-
-
class
semilattices.
LabeledSparseVertex
(obj=None, *args, **kwargs)[source]¶ A vertex that is
LabeledVertex
andSparseVertex
.
-
class
semilattices.
SparseCoordinateVertex
(obj=None, *args, **kwargs)[source]¶ A vertex that is
CoordinateVertex
andSparseVertex
.
-
class
semilattices.
LabeledCoordinateVertex
(obj=None, *args, **kwargs)[source]¶ A vertex that is
LabeledVertex
andCoordinateVertex
.In order to be “comparable”, a Labeled Vertex must be assigned a
LabeledVertex.label
(i.e. scalar or anything ‘<’ comparable). Comparison between twoLabeledCoordinateVertex
is done first according to theLabeledVertex.label
, then according to theCoordinateVertex.coordinates
. Vertices with missingLabeledVertex.label
are always considered to be smaller than vertices with labels. If both the vertices are not “comparable”, i.e. are not assigned labels, then compare only byCoordinateVertex.coordinates
.To make sorted data structures robust to the user changing the
LabeledVertex.label
, the data structure containing the element should be used to update the label of the vertices.Keyword Arguments: - label – label for the vertex. Default is
None
- coordinate (CoordinateDict) – semilattice coordinate
- label – label for the vertex. Default is
-
class
semilattices.
SparseLabeledCoordinateVertex
(obj=None, *args, **kwargs)[source]¶ A vertex that is
LabeledVertex
,CoordinateVertex
andSparseVertex
.
-
class
semilattices.
Semilattice
(*args, **kwargs)[source]¶ An (order-) semilattice is a rooted connected directed acyclic graph resembling the structure of a tree, i.e. it has a depth/levels. However, unlike a tree, each vertex can have multiple parents.
We denote the in-vertex neighbors of a vertex in the graph as
parents
and the out-vertex neighbors of a vertex in the graphchildren
.ancestors
anddescentants
follow the same terminology.-
__init__
(*args, **kwargs)[source]¶ - Optional Args:
- semilattice (Semilattice): a semilattice to cast from
Keyword Arguments: - VertexConstructor (class) – a subclass of
SemilatticeVertex
(default:SemilatticeVertex
) - VertexSetConstructor (class) – a container class defining the data structure
containing vertices (default:
set
)
-
__eq__
(other)[source]¶ Checks whether two semilattices have the same structure and
VertexConstructor
-
__ge__
(other, NotImplemented=NotImplemented)¶ Return a >= b. Computed by @total_ordering from (not a <= b) or (a == b).
-
__iter__
()[source]¶ The default iterator iterates over the datastructure defined to contain the vertices
-
__ior__
(other)[source]¶ Returns the in-place union of two graphs.
For the elements in the intersection of
self
andother
it always copies vertices fromself
.Parameters: - self (Semilattice) – first graph
- other (Semilattice) – second graph
Returns: (
Semilattice
) – the union ofself
andother
-
__or__
(other)[source]¶ Returns the non-in-place union of two graphs.
For the elements in the intersection of
self
andother
it always copies vertices fromself
.Parameters: - self (Semilattice) – first graph
- other (Semilattice) – second graph
Returns: (
Semilattice
) – the union ofself
andother
-
__iand__
(other)[source]¶ Returns the in-place intersection of two graphs.
Elements are always retained from
self
.Parameters: - self (Semilattice) – first graph
- other (Semilattice) – second graph
Returns: (
Semilattice
) – the intersection ofself
andother
-
__and__
(other)[source]¶ Returns the non-in-place intersection of two graphs.
Elements are always retained from
self
.Parameters: - self (Semilattice) – first graph
- other (Semilattice) – second graph
Returns: (
Semilattice
) – the intersection ofself
andother
-
class
Properties
(VertexConstructor, VertexSetConstructor, VertexSetConstructorKwargs)¶ -
VertexConstructor
¶ Alias for field number 0
-
VertexSetConstructor
¶ Alias for field number 1
-
VertexSetConstructorKwargs
¶ Alias for field number 2
-
-
VertexConstructor
¶ Class constructor of the vertices in the semilattice.
Type: class
-
VertexSetConstructor
¶ Class constructor for sets of vertices in the semilattice.
Type: class
-
VertexSetConstructorKwargs
¶ Arguments to pass into a Vertex Set Constructor. This is particularly used when, for example, labels are set for vertex sets that are sorted
Type: class
-
append
(v, other)[source]¶ Appends a semilattice to the vertex v of
self
Parameters: - v (Vertex) – vertex to which append the semilattice
- other (Semilattice) – semilattice to be appended
-
children_adjacency_mat
¶ Adjacency matrix built traversing children
Returns: - Adjacency matrix (type self.adjacency_mat_type) built by
- traversing the parents
-
delete_vertex
(deletion_target)[source]¶ Deletes a vertex from the semilattice.
Parameters: deletion_target ( SemilatticeVertex
) – vertex to be deletedReturns: - (
set
) – set containing all the vertices removed from the - semilattice in the process of remoing
deletion_target
- (
-
intersection
(other)[source]¶ In-place intersection of two graphs.
Elements are always retained from
self
.Parameters: - self (Semilattice) – first graphs
- other (Semilattice) – second graphs
-
is_comparable_to
(other)[source]¶ Whether the semilattice is comparable to
other
.Two semilattices are comparable if their
VertexConstructor
are the same.
-
iterator
(start_vertex=None, iter_attribute='children', iter_type=<class 'semilattices._iterables.BreadthFirstSemilatticeIterable'>)[source]¶ Returns an iterator for the semilattice.
See
SemilatticeIterable
for more details.
-
multiply
(other)[source]¶ Inplace product of the two semilattices
A product of two semilattices results in an increased semilattice where from each vertex of
self
starts a fullother
semilattice. The cost of this operation is \(\mathcal{O}(n_1 n_2)\) in the worst case.Parameters: - self (Semilattice) – first semilattice
- other (Semilattice) – second semilattice
-
new_vertex
(**kwargs)[source]¶ Adds a new child to
parent
along the directionedge
Keyword Arguments: - edge (int) – edge for child
- parent (Vertex) – vertex to which to add a child
- Optional Keword Args:
new_vertex (Vertex): the new vertex, constructed on the outside **kwargs: keyword arguments for the construction of the new vertex,
to be passed to the vertex constructor
Returns: (
SemilatticeVertex
) – the added vertexRaises: InvalidVertex
– if the vertex is not an instance ofVertex
ChildAlreadyExists
– ifvertex
already has aedge
child.
-
order
()[source]¶ Same as
cardinality
-
parents_adjacency_mat
¶ Adjacency matrix built traversing parents
Returns: - Adjacency matrix (type self.adjacency_mat_type) built by
- traversing the parents
-
properties
¶ Properties of the semilattice that define construction of a new empty semilattice. This quantity it essentially ‘immutable’. The user should not try to modify self._properties (undefined behavior for this code)
-
random_vertex
()[source]¶ Return a random vertex from the semilattice.
Returns: ( SemilatticeVertex
) – random vertex
-
root
¶ The root vertex
Type: SemilatticeVertex
-
union
(other, start_vertex_self=None, start_vertex_other=None)[source]¶ In-place union of two graphs.
For the elements in the intersection of
self
andother
it always copies vertices fromself
.Parameters: - self (Semilattice) – first graph
- other (Semilattice) – second graph
- start_vertex_self (Vertex) – vertex of
self
from which to start the union - start_vertex_other (Vertex) – vertex of
other
from which to start the union
-
-
class
semilattices.
CoordinateSemilattice
(*args, **kwargs)[source]¶ A semilattice with pre-defined dimension.
A (meet order-) semilattice is a partially-ordered set of vertices \((\mathcal{S}, \le)\), where the binary relation \(\le\) defines a meet between \(x\) and \(y\) \(\in \mathcal{S}\) in the following way: \(\forall x,y \in \mathcal{S}, x \le y \equiv x \wedge y = x\). A semilattice has the property that the infimum/meet/greatest lower bound \(z = (x \wedge y) \in \mathcal{S}\). The root vertex is the infimum \(z\) of all vertices in the semilattice.
-
__init__
(*args, **kwargs)[source]¶ - Optional Args:
- semilattice (Semilattice): a semilattice to cast from
Keyword Arguments: - dims (int) – semilattice dimension (maximum number of children per vertex)
- VertexConstructor (class) – a subclass of
CoordinateVertex
(default:CoordinateVertex
) - VertexSetConstructor (class) – a container class defining the data structure
containing vertices (default:
SortedSet
) - l1_vertices_partition (bool) – whether to keep track of the
\(\ell^1\) partition of vertices (default:
True
) - l1_frontier_partition (bool) – whether to keep track of the
\(\ell^1\) partition of the frontier (default:
True
)
-
class
Properties
(VertexConstructor, VertexSetConstructor, VertexSetConstructorKwargs, l1_vertices_partition_flag, l1_frontier_partition_flag, l1_childless_partition_flag, dims)¶ -
VertexConstructor
¶ Alias for field number 0
-
VertexSetConstructor
¶ Alias for field number 1
-
VertexSetConstructorKwargs
¶ Alias for field number 2
-
dims
¶ Alias for field number 6
-
l1_childless_partition_flag
¶ Alias for field number 5
-
l1_frontier_partition_flag
¶ Alias for field number 4
-
l1_vertices_partition_flag
¶ Alias for field number 3
-
-
childless
¶ Childless set of vertices in the semilattice.
The childless vertices are the set of vertices which don’t have any children. It is a subset of the frontier.
-
draw
(ax=None, **kwargs)[source]¶ Draw the semilattice.
Parameters: ax – matplotlib axes
Keyword Arguments:
-
frontier
¶ Set of vertices in the frontier of the semilattice.
The frontier is defined to be the set of vertices which don’t have all the children.
-
is_comparable_to
(other)[source]¶ Checks if
self
is comparable toother
Parameters: other (Vertex) – vertex to check if self is comparable to
-
max_coordinates
¶ Returns the coordinates of the greatest coordinates in each effective dimension of the semilattice
-
max_l1_norm
¶ Returns the maximum l1 norm of any coordinate in the semilattice
-
modify_dims
(**kwargs)[source]¶ Modify the dimension
dims
of the semilattice.- **kwargs:
- add_dims (uint): (positive #) dimensions to add to
dims
(optional) percent_increase (uint): (positive #) percent to expanddims
. Floor rounding takes place. (optional)
-
num_potential_children_of
(parent)[source]¶ Number of dimensions where
parent
can have childrenParameters: parent ( CoordinateSemilatticeVertex
) – parent vertexReturns: ( int
) – number of dimensions whereparent
can have children
-
potential_children_edges_of
(parent)[source]¶ List of dimensions where
parent
can have childrenParameters: parent ( CoordinateSemilatticeVertex
) – parent vertexReturns: ( list
) – list of dimensions whereparent
can have children
-
-
class
semilattices.
DecreasingCoordinateSemilattice
(*args, **kwargs)[source]¶ A DecreasingCoordinateSemilattice is a semilattice \((\mathcal{S}\,\le)\) that is closed under meets, that is it is decreasing: \(x \le y \implies x \in \mathcal{S}\). A decreasing semilattice is often called ‘downward-closed, or ‘admissible’ when the semilattice is interpreted as a multiindex set parameterizing quadrature or interpolation problems
Parameters: - dims (int) – semilattice dimension (maximum number of children per vertex)
- VertexConstructor (class) – type of vertices in the semilattice.
It must extend
SparseCoordinateVertex
-
class
Properties
(VertexConstructor, VertexSetConstructor, VertexSetConstructorKwargs, l1_vertices_partition_flag, l1_frontier_partition_flag, l1_childless_partition_flag, dims, l1_admissible_frontier_partition_flag)¶ -
VertexConstructor
¶ Alias for field number 0
-
VertexSetConstructor
¶ Alias for field number 1
-
VertexSetConstructorKwargs
¶ Alias for field number 2
-
dims
¶ Alias for field number 6
-
l1_admissible_frontier_partition_flag
¶ Alias for field number 7
-
l1_childless_partition_flag
¶ Alias for field number 5
-
l1_frontier_partition_flag
¶ Alias for field number 4
-
l1_vertices_partition_flag
¶ Alias for field number 3
-
-
admissible_frontier
¶ The set of fontier vertices with admissible an admissible child(ren). Sorted by lexicographic ordering of the vertices.
-
draw
(ax=None, **kwargs)[source]¶ Draw the semilattice.
For additional keyword arguments see
CoordinateSemialattice.draw()
.Parameters: ax – matplotlib axes Keyword Arguments: mark_admissible_frontier (bool) – if set to true marks the admissible frontier nodes
-
l1_admissible_frontier_partition
¶ A partition of the admissible frontier by l1 norm
-
num_potential_children_of
(parent)[source]¶ Return number of all possible edges for new children of a parent
-
random_potential_children_edge_of
(parent)[source]¶ Return a random possible edge for a new child for a parent When one need a random potential children_edge, the user should use this function,
-
class
semilattices.
SortedCoordinateSemilattice
(*args, **kwargs)[source]¶ A SortedCoordinateSemilattice is a semilattice with Labeled vertices.
The elements of the SortedCoordinateSemilattice are sorted by the labels in a heap. One can add and remove labeled vertices in the lattice, and the ordering is maintained by the heap. SortedCoordinateSemilattice relies on the LabeledVertex and MixedSortedContainer data structures.
-
class
Properties
(VertexConstructor, VertexSetConstructor, VertexSetConstructorKwargs, l1_vertices_partition_flag, l1_frontier_partition_flag, l1_childless_partition_flag, dims, label_keys, default_label_key, data_keys)¶ -
VertexConstructor
¶ Alias for field number 0
-
VertexSetConstructor
¶ Alias for field number 1
-
VertexSetConstructorKwargs
¶ Alias for field number 2
-
data_keys
¶ Alias for field number 9
-
default_label_key
¶ Alias for field number 8
-
dims
¶ Alias for field number 6
-
l1_childless_partition_flag
¶ Alias for field number 5
-
l1_frontier_partition_flag
¶ Alias for field number 4
-
l1_vertices_partition_flag
¶ Alias for field number 3
-
label_keys
¶ Alias for field number 7
-
-
draw
(ax=None, **kwargs)[source]¶ Draw the semilattice.
For additional keyword arguments see
CoordinateSemialattice.draw()
.Parameters: ax – matplotlib axes Keyword Arguments: color (bool) – if set to labels
colors with respect to thelabel
of the vertex
-
sorted
¶ Returns the sorted labeled vertices based on the default label
Warning
It will iterate ONLY over the vertices that have been assigned a label. A warning will be raised if some of the vertices have not been labeled.
-
sorted_frontier
¶ Returns the sorted frontier based on the default label
Warning
It will iterate ONLY over the vertices that have been assigned a label. A warning will be raised if some of the vertices have not been labeled.
-
update_data
(vertex, **kwargs)[source]¶ Update metadata of a vertex
Parameters: vertex (LabeledVertex) – vertex to label Keyword Arguments: keyword arg should be metadata of the vertex (each) –
-
update_labels
(vertex, **kwargs)[source]¶ Update label(s) of a vertex
Parameters: vertex (LabeledVertex) – vertex to label Keyword Arguments: keyword arg should be a label of the vertex (each) –
-
class
-
class
semilattices.
SortedDecreasingCoordinateSemilattice
(*args, **kwargs)[source]¶ -
class
Properties
(VertexSetConstructorKwargs, l1_childless_partition_flag, default_label_key, l1_frontier_partition_flag, VertexSetConstructor, l1_vertices_partition_flag, label_keys, l1_admissible_frontier_partition_flag, dims, data_keys, VertexConstructor)¶ -
VertexConstructor
¶ Alias for field number 10
-
VertexSetConstructor
¶ Alias for field number 4
-
VertexSetConstructorKwargs
¶ Alias for field number 0
-
data_keys
¶ Alias for field number 9
-
default_label_key
¶ Alias for field number 2
-
dims
¶ Alias for field number 8
-
l1_admissible_frontier_partition_flag
¶ Alias for field number 7
-
l1_childless_partition_flag
¶ Alias for field number 1
-
l1_frontier_partition_flag
¶ Alias for field number 3
-
l1_vertices_partition_flag
¶ Alias for field number 5
-
label_keys
¶ Alias for field number 6
-
-
class
-
semilattices.
create_lp_semilattice
(dims, norm, p=1, weights=None, SemilatticeConstructor=<class 'semilattices._decreasingcoordinatesemilatticebase.DecreasingCoordinateSemilattice'>, **kwargs)[source]¶ Create the semilattice of all vertices in the \(\ell^p\) spherical sector of a given norm
For a particular
norm
value, \(0\leq p\leq \infty\), and optional anisotropic weighting for the \(p\) norm, this function creates a semilattice of all vertices enclosed in the \(\ell^p\) spherical sector of the given norm size, with optional anisotropic dimension dependence. The vertices form a decreasing coordinate semilattice.Parameters: - dim (int) – The dimension of the semilattice
- norm (float) – norm of the \(\ell^p\) spherical sector enclosing all vertices of the output semilattice
- p (int) – The \(p\) in \(\ell^p\)
- weights (
SpaceWeightDict
) – A weighting on the \(\ell^p\) norm \(\|x \|_{\ell^p_W} = (\sum_{i=1}^{dim} w_i | x_i |^p)^{1/p}\). Each dictionary key is the dimension \(i\), while while each corresponding value is \(w_i\). Weights must be>1
. Missing values are considered 1. - SemilatticeConstructor (
DecreasingCoordinateSemilattice
) – derived subclass ofDecreasingCoordinateSemilattice
defining the type of semilattice to return - **kwargs – additional keyword arguments to be passed to the
SemilatticeConstructor
specified. See the specific semilattices for the list of keyword arguments that may be provided.
Returns: (
DecreasingCoordinateSemilattice
) – The constructed semilattice
-
semilattices.
permute
(sl, p)[source]¶ Creates a new semilattice with permuted dimensions
We assume the semilattice
sl
to have dimensions(0, 1, 2)
. Given the permutation(2, 0, 1)
this function will output a semilatticesl1
with the following propery: beingv
a vertex ofsl
andv1
the corresponding vertex ofsl1
, thenv1.children[0] == v.children[2]
,v1.children[1] == v.children[0]
,v1.children[2] == v.children[1]
, where the vertices aredeepcopy
of one-another.Parameters: - sl (
CoordinateSemilattice
) – input semilattice. - p (
Iterable
) – permuted dimensions.
- sl (